Building a Bolt Slack Bot: A Straightforward Starter-Kit Guide
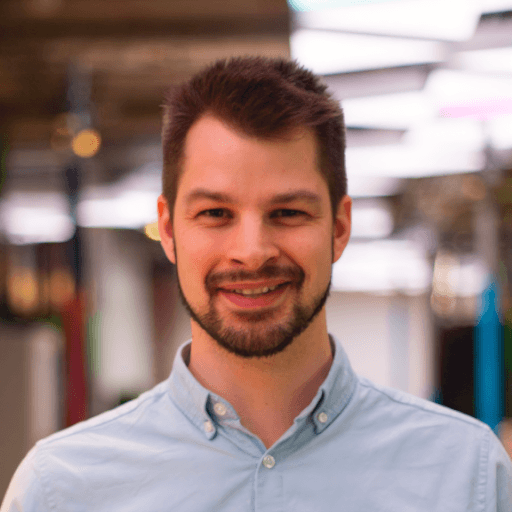
Richard Casemore - @skarard
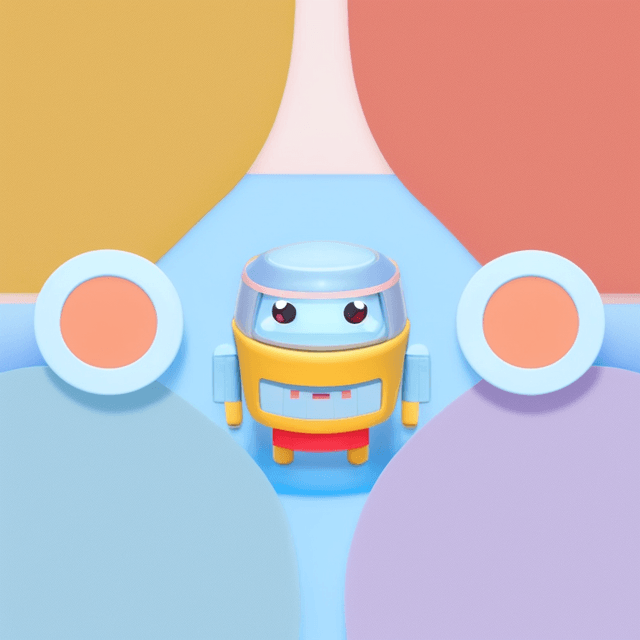
Introduction
In today's fast-paced tech world, Slack bots have become essential tools for automating tasks and enhancing user experience. Whether you're a seasoned developer or a curious newcomer, building your own Slack bot might seem like a daunting task. The good news is, it doesn't have to be!
This guide, the first part of a two-part series, will walk you through creating a bot on Slack, step-by-step. We'll start from the basics, setting up the environment, understanding the code, and firing up our very own Slack bot. We’ll explain each stop on our journey, breaking everything down into digestible chunks that anyone can understand!
In part two, we'll make our bot smarter! We'll show you how to connect your bot to Langchain and Notion, transforming it into an intelligent, conversational agent that can retrieve information from a Notion database, making it an invaluable study aid capable of answering your queries.
So let your curiosity drive you, roll up those sleeves, and get ready to embark on an exciting journey into the world of bot development!
Prerequisites
To follow this guide on building a Slack bot, you'll need the following installed on your system:
- Git: We will be cloning the project repository using Git. If Git isn't installed on your system, refer to the Git documentation for installation instructions.
- Node.js: Our bot will be running on a Node.js environment. If Node.js isn't installed yet, you can get it from the Node.js download page.
- A Slack workspace: To deploy and test our bot, you need a Slack workspace. If you don't have one, you can create one for free on Slack's website.
We'll cover the necessary basics of TypeScript, using Slack's Bolt framework, and setting up your Slack workspace as we progress through the article. Whether you're an experienced developer or just starting out on your coding journey, this guide will provide you with the knowledge and tools you need to create an efficient Slack bot!
Setting Up Your Workspace
To get started, you first need to clone the repository which contains the necessary boilerplate code for our Slack bot. You can do this using the following command:
git clone https://github.com/metalumna/bolt-sdk-slack-bot-starter-kit.git
After cloning, move into the project directory:
cd bolt-sdk-slack-bot-starter-kit
Inside the directory, you should find all the essential files for our bot's operation.
The next step is to install all necessary dependencies for our project. We'll do this by running:
npm install
Now that your workspace is all set up, the next thing you need to do is create your .env
file to store all your environment variables.
In the root of the project directory, you'll find a file named .env.example
. This file contains all the environment variables that are necessary for the operation of the bot. You can simply make a copy of this file and rename the copy to .env
like so:
cp .env.example .env
Once you've done this, you're ready to start populating the .env
file with your own values. In the next section we will find all the details to fill in the Slack's Signing Secret, Bot Token, App Token.
Configuring Your Slack Account
In order to create a Slack bot, we need to set up some configurations on the Slack apps website as well. The following steps will guide you through this process:
-
Create a new Slack App: Head to Slack API's app page and click on "Create New App". Here, name your App, select the workspace where this bot would work on, and then click "Create App".
-
Configure Permissions: Under "Features" option in the side menu, click on "OAuth & Permissions". Scroll down to the "Scopes" section. Add the necessary bot token scopes based on what commands your bot would need. For our simple echo bot, adding
chat:write
, will suffice. -
Enable Direct Messages: Under "Features" option in the side menu, click on "App Home". Scroll down to the "Show Tabs: section. Ensure the Messages Tab toggle is on, then enable the tickbox "Allow users to send Slash commands and messages from the messages tab".
-
Configure Socket: Under "Settings" option in the side menu, click on "Socket Mode". Switch the Enable Socket Mode toggle to the on position. You will be asked to add
connections:write
scope to an app-level token, you can name this token "Socket". Then press Generate, copy the app-level token toSLACK_APP_TOKEN
. -
Install App to Workspace: Once you've configured your socket, Under the "settings option in the side menu, click on "Install App". Install the app on to your workspace, then there is one more step before we gather up all the tokens.
-
Configure Events: Under "Features" option in the side menu, click on "Event Subscriptions". Toggle Enable Events to be on. Open up the "Subscribe to bot events" menu, then "Add Bot User Event". Adding the
message.im
event will be enough for our echo bot. Make sure to press "Save Changes" on the bottom right of the page. Go back to the "Install App" page (Under the "settings option in the side menu, click on "Install App"), this time press "Reinstall to Workspace". Copy the Bot User OAuth Token toSLACK_BOT_TOKEN
-
Signing Secret In the side menu, under "Settings", click on "Basic information". Scroll down to "App Credentials" section and locate "Signing Secret". Hit "Show" to reveal the secret and copy it to
SLACK_SIGNING_SECRET
.
That's it! Slack is configured for your Slack bot. Remember to never share any of your tokens and especially not the signing secret.
Running and Testing the Bot
After we've configured all the settings in both our local environment and Slack workspace, it's time to finally run our Slack bot server!
To get your bot up and running, navigate back to your command line, ensure you're in the correct directory, and type the following command:
npm run dev
This command activates the server in development mode. The server is configured to restart automatically every time there's a change to any of our files, thanks to nodemon.
After running this command, you should see the console print out "[server] Bolt is running" if everything is set up correctly. This means the bot is now live and listening for events!
Now, head over to the Slack workspace you installed your app to. Start a conversation with your Bot User. Send any text message, and voila! Your bot should immediately reply with "Echo: " followed by your message. And there you have it, your bot is up and running!
Remember, the code for our bot as it stands is just the basics.
Understanding the Code
/src/config.ts
- Configuration for Environment Variables
The very first part in our server code deals with setting up our environment variables. These are global variables the server can reference to make decisions. For our server, we have them housed in a .env
file. The contents of the .env
file is loaded into process.env
when we call import dotenv/config
.
We've defined a class EnvVars
which contains default values and defines the TypeScript type of each variable, in this example PORT
s are of the number
type and TOKEN
s & SECRET
are of the string
type. This allows for improved TypeScript typing and code compeltion when developing our application.
class EnvVars {
SLACK_SIGNING_SECRET = "";
SLACK_BOT_TOKEN = "";
SLACK_APP_TOKEN = "";
SLACK_PORT = 8080;
}
If you add a new environment variable to the .env
file, you will need to add its name and a default value to the EnvVars
class.
Then we go ahead to process all these environment variables to ensure they exist, if not we print an error and exit the process. This means the environment variables are defined and typed correctly before we can start the application. The bonus here is that we notified of which environment variables are not set correctly.
/src/bolt.ts
- Initializing the Bolt App
The Slack API is wrapped by Slack's Bolt SDK, a powerful framework that makes it simple to develop interactive apps, to help us execute functions when certain events or triggers happen in Slack. In our bolt.ts
file, we initialize the instance of Bolt.
import config from "./config";
import { App } from "@slack/bolt";
export const boltApp = new App({
signingSecret: config.SLACK_SIGNING_SECRET,
token: config.SLACK_BOT_TOKEN,
appToken: config.SLACK_APP_TOKEN,
socketMode: true,
});
We import the config
file, which contains the values of our environment variables.
In the App
constructor, we pass in an object of various parameters such as Signing Secret, Bot Token, App Token, etc. - all these are HTTPS endpoint configurations necessary for the Slack API to function properly.
The socketMode
option determines whether or not the app runs on Socket Mode. Socket Mode makes your app listen to the Slack API via WebSocket connections and is a replacement for the older Events API.
We then set up a Listener for our bot. It listens to all message
events and can respond to messages. This is a simple implementation in which our bot responds with an echo of every message it receives. Your bot can essentially listen to all sorts of events and you can customise unique responses for each.
boltApp.message(async ({ message, say }) => {
if (message.subtype === undefined || message.subtype === "bot_message") {
await say(`Echo: ${message.text}`);
}
});
/src/index.ts
- Running the Server
Finally, after setting up our environment variables, initializing configuration, and creating our bot, we come to the final part of setting everything into motion which happens in index.ts
.
import config from "./config";
import { boltApp } from "./bolt";
(async () => {
boltApp
.start(config.SLACK_PORT)
.then(() => console.log("[server] Bolt is running"));
})();
There is an asynchronous function wherein we start the Bolt app. We use .start()
for Bolt App to start the servers. This function listens for incoming WebSocket connections.
The console.log
outputs are useful for us to understand if the servers were started successfully. If you see "[server] Bolt is running" in your terminal, it means the server was started and is listening to WebSocket connections.
What's Next?
With all the hard work behind us, we're ready to turn our simple Slack bot into something more advanced and useful. The next step? Integrating our bot with LangChain and Notion for a conversational knowledge base!
In the next article, we'll walk through how to supercharge our Slack bot by connecting it to a powerful Large Language Model. By doing so, we can enable our bot to understand natural language, carry out tasks, answer questions, and add much more functionality.
Imagine creating a bot that not only echoes messages but one that can manage tasks, answer queries, and interact with users in a way that goes beyond predefined commands. The possibilities are endless and it all begins with integration!
Next Guide: Unleashing the Potential of a Bolt Slack Bot: A Thorough Guide to Integrating Langchain and Notion